Table of contents
- Introduction
- Getting Started
- Prerequisites
- How to get B2B emails with
Work Email Lookup API
Endpoint - How to get personal emails with
Personal Email Lookup API
Endpoint - How to get personal numbers with
Personal Number Lookup API
Endpoint - Summary
Introduction
It is a common use case to reach out to a Professional Social Network profile for hiring or sales. Professional Social Network inMail is limited because you have to pay a significant price per outreach. You will probably need an external solution.
Some tools help you fetch a work email from any Professional Social Network profile. But more often or not, these are tools with a user interface intended for end-users. If you're seeking a user interface tool, this is not the article for you.
In this article, you'll learn how to use Proxycurl's Contact API to get verified B2B emails and personal numbers from Professional Social Network profiles programmatically.
This article is for teams with software engineers looking to implement an Email Finding API within their product or business workflow, so you can fetch B2B emails at scale, programmatically. With Proxycurl's Contact API, You can get verified work emails of any Professional Social Network profile.
On top of just work emaills, you can also get the following data with Proxycurl API:
- Personal emails
- Personal number
- Social media accounts
In addition, emails returned by our API are:
- Verified with a 95%+ deliverability guarantee
- Always fresh - even if the profile has changed a job, the API can fetch his latest work email.
Getting Started
This tutorial will show you how to query the Contact API endpoints in Node.js using express
and axios
. This tutorial aims to show you how easy it is to use the Contact API and get your results.
Steps we'll cover
- How to get B2B emails with Work Email Lookup API Endpoint.
- How to get personal emails with Personal Email Lookup API Endpoint.
- How to get personal numbers with Personal Number Lookup API Endpoint.
Prerequisites
In your preferred working directory, initialize a new project by running:
npm init -y
Next, run the following command to install the packages to bootstrap your application:
npm install express axios dotenv
express
- a minimal and flexible Node.js framework to bootstrap our server.axios
- a lightweight HTTP-based data fetching library to query the Contact API endpoints.dotenv
- lets us load environment variables seamlessly into the node.js process.
To begin using Proxycurl's Contact API, you'll need an API KEY to make requests to the various endpoints. Sign up here to get started.
With your API KEY ready (you can copy it from API Key and billing tab in your dashboard), create a .env
file in your project root directory and add the following code to it:
API_KEY = '<your_api_key>'
Next, in your project root directory create another file index.js
and add the following code to it:
import express from 'express';
import dotenv from 'dotenv';
import axios from 'axios';
const port = 8000;
const app = express();
dotenv.config();
app.listen(port, () => {
console.log(`App connected successfully on port: ${port}`);
});
Next, add "type": "module"
in package.json
to let Node.js know we're writing ES6 code and edit the script
field to the following:
"scripts": {
"dev": "node index.js"
}
Run npm run dev
in the terminal and the following should be logged to the console if everything went smoothly:
App connected successfully on port: 8000
How to get B2B emails with Work Email Lookup API
Endpoint
The Work Email Lookup Endpoint lets you get the work email address of a Professional Social Network profile.
Endpoint URL
The endpoint URL to get a Professional Social Network profile work email is https://nubela.co/proxycurl/api/Professional Social Network/profile/email
, and every request you make to the endpoint costs 3
credits.
In this tutorial, We'll explore two methods by which you can leverage the URL to get a person's work email in your applications.
With webhooks
The Work Email Lookup Endpoint may not return results immediately since we need to perform real-time heuristics to fetch and verify the email. The endpoint requires a mandatory parameter: Professional Social Network_profile_url
. This is the Professional Social Network profile URL of the person whose work email we're looking for.
Alternatively, by adding an optional callback_url
parameter to the request, we can create a webhook with Webhook.site to listen to the result once it's available. Webhook.site gives us a randomly generated URL to listen to, so whenever we query the work email endpoint, the response, which is an object in the following format: {'email': ..., 'status': ...}
, will be sent to your Webhook.site custom URL.
To get started, visit Webhook.site to get your custom URL. The webpage should look like the below:
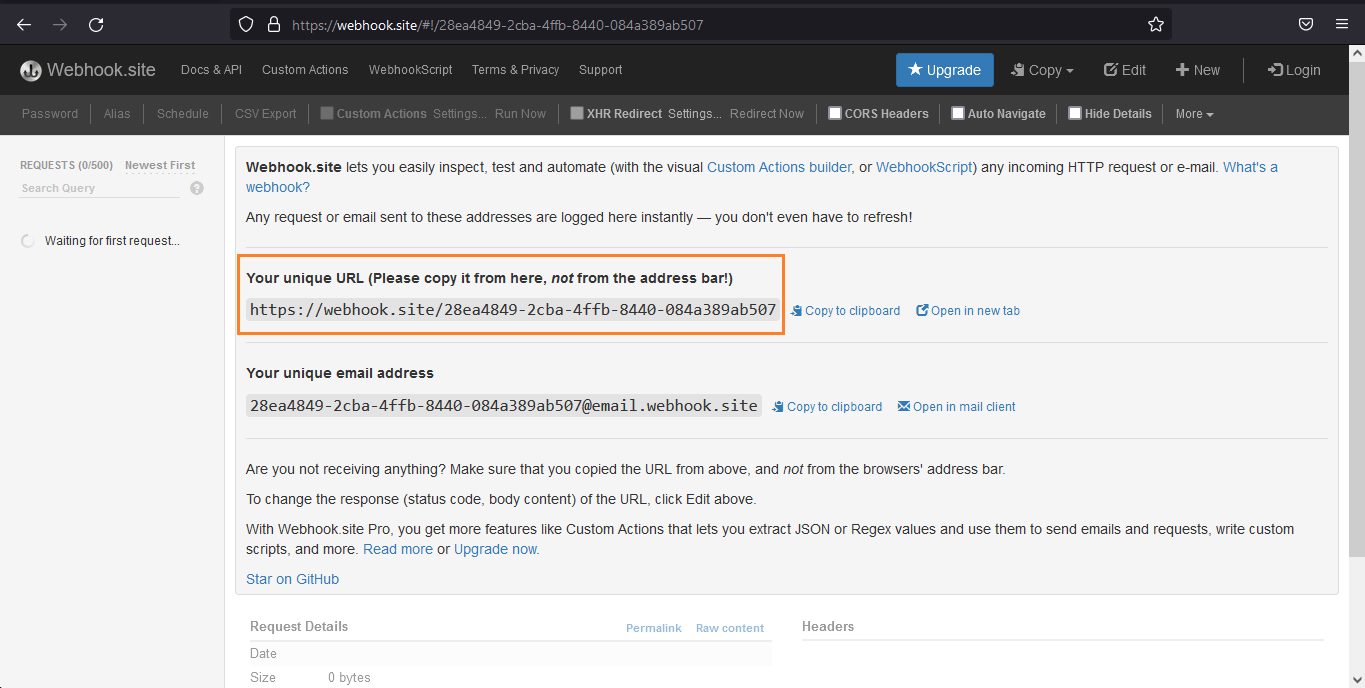
Copy the URL and add it to the WEBHOOK_URL
variable in your code like so:
const WEBHOOK_URL = '<your_webhook.site_url>'
Here's the complete code for this example:
// index.js
import express from 'express';
import dotenv from 'dotenv';
import axios from 'axios';
const port = 8000;
const app = express();
dotenv.config();
const WORK_EMAIL_ENDPOINT = 'https://nubela.co/proxycurl/api/Professional Social Network/profile/email';
const Professional Social Network_PROFILE_URL = 'https://www.professionalsocialnetwork.com/in/williamhgates/';
const WEBHOOK_URL = 'https://webhook.site/18fa951e-8cd1-4345-847d-e7ae329e147c'
const workEmailConfig = {
url: WORK_EMAIL_ENDPOINT,
method: 'get',
headers: {'Authorization': 'Bearer ' + process.env.API_KEY},
params: {
Professional Social Network_profile_url: Professional Social Network_PROFILE_URL,
callback_url: WEBHOOK_URL
}
};
const getWorkEmail = async () => {
try {
return await axios(workEmailConfig);
}
catch (err) {
throw new Error(err)
}
}
const email = await getWorkEmail();
console.log('Work Email:', email.data.email_queue_count);
app.listen(port, () => {
console.log(`App connected successfully on port: ${port}`);
});
Now if you visit Webhook.site the response of the query can be seen as shown below:
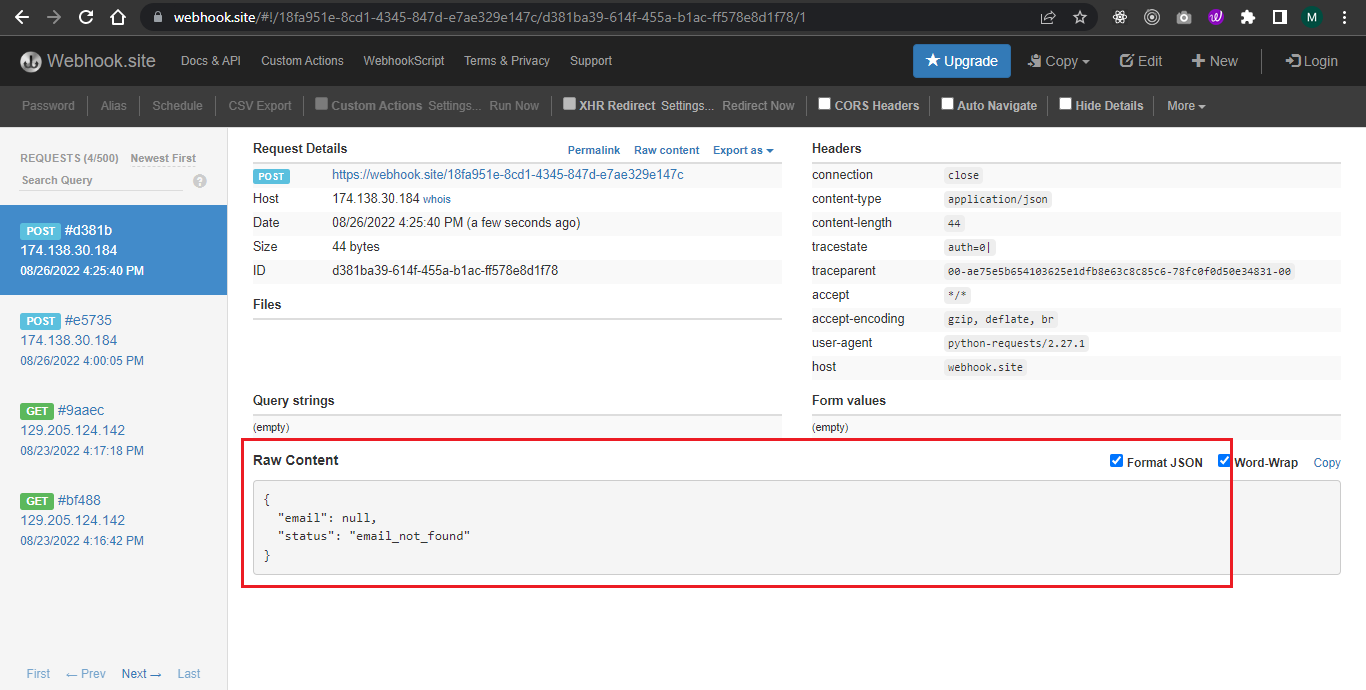
In our example, the Professional Social Network profile we searched for doesn't have a work email, so the email
and status
fields in the response returned null
and email_not_found
, respectively.
With Dashboard logs
What's interesting to note is that Proxycurl automatically logs every response to the dashboard whenever the Work Email API Endpoint is queried. So log on to your dashboard and navigate the Work Email Lookup Logs tab to see it in action.Here's what it looks like with the logs of our previous queries:
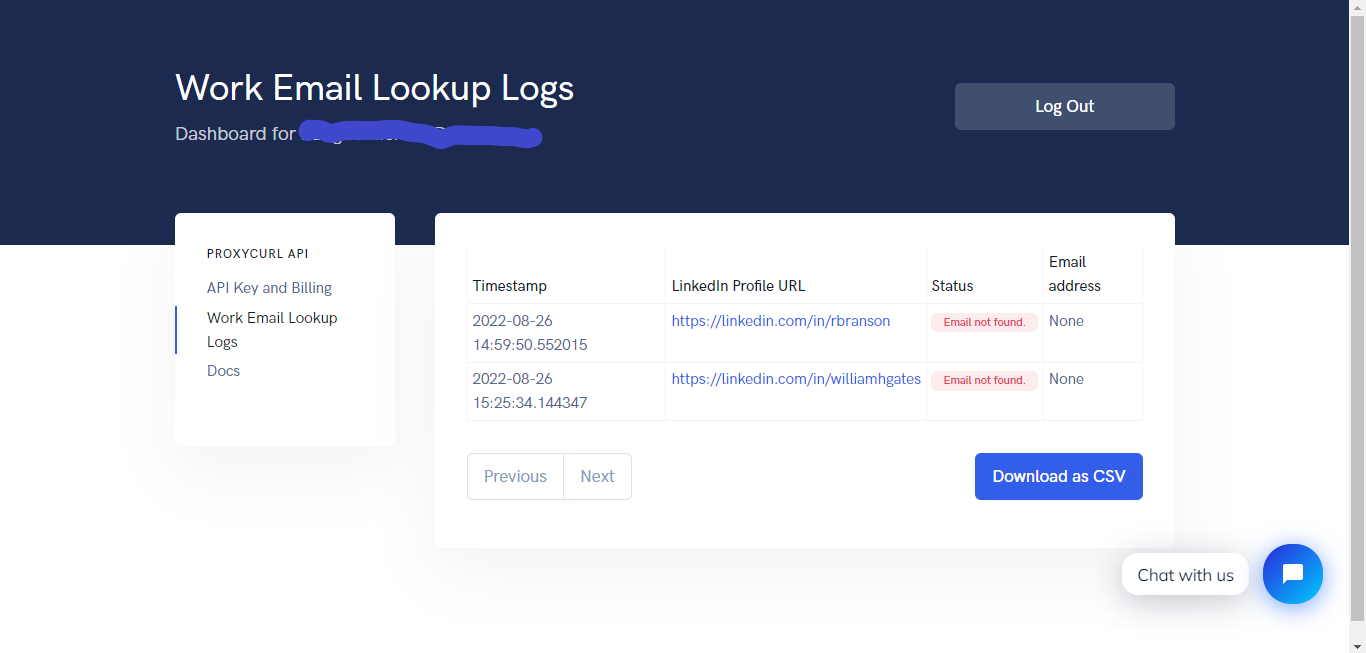
The dashboard logs also provide additional information, such as the timestamp of the query and the Professional Social Network profile URL we're searching for, which is pretty awesome. You can also export the logs by clicking the Download as CSV
button on your dashboard.
How to get personal emails with Personal Email Lookup API
Endpoint
With the Personal Email Lookup API Endpoint you can get the list of personal emails belonging to a Professional Social Network profile at the cost of 1
credit per every email returned.
Endpoint URL
The endpoint for the personal email lookup is https://nubela.co/proxycurl/api/contact-api/personal-email
.
Parameters
The endpoint requires a mandatory parameter: Professional Social Network_profile_url
, which is the Professional Social Network profile URL of the person whose personal email we're looking for, and an optional email_validation
parameter to specify if we want an additional validation of the emails found in the profile (this comes at an additional cost of 1
credit per every email that is found). The email_validation
parameter accepts a string value of include
. Defaults to exclude
.
// axios config example
params: {
email_validation: 'include'
}
Response
The personal email lookup returns an object with two properties: an emails
field containing a list of personal emails of the given Professional Social Network profile, and an invalid_emails
field containing invalid emails of a Professional Social Network profile. If the email_validation
parameter isn't specified, the invalid_emails
field will return an empty array.
The demo below shows how easy it is to use the endpoint:
// index.js
{ ... }
const PERSONAL_EMAIL_ENDPOINT = 'https://nubela.co/proxycurl/api/contact-api/personal-email';
const Professional Social Network_PROFILE_URL = 'https://professionalsocialnetwork.com/in/steven-goh-6738131b';
const personalEmailConfig = {
url: PERSONAL_EMAIL_ENDPOINT,
method: "get",
headers: { Authorization: "Bearer " + process.env.API_KEY },
params: {
Professional Social Network_profile_url: Professional Social Network_PROFILE_URL,
email_validation: "include",
},
};
const getPersonalEmail = async () => {
try {
return await axios(personalEmailConfig);
} catch (err) {
throw new Error(err);
}
};
const email = await getPersonalEmail();
console.log("Personal Email:", email.data);
{ ... }
Which logs the following response to the console:
Personal Email: {
emails: [ '[email protected]' ],
invalid_emails: [ '[email protected]' ]
How to get personal numbers with Personal Number Lookup API
Endpoint
With the Personal Number Lookup API Endpoint you can get the list of personal numbers belonging to a Professional Social Network profile at the cost of 1
credit per every contact number returned.
Endpoint
The endpoint for the personal number lookup is: https://nubela.co/proxycurl/api/contact-api/personal-contact.
Parameters
The personal number lookup endpoint accepts a single required parameter: Professional Social Network_profile_url
. This is the URL of the Professional Social Network profile whose number we're looking for.
Response
The endpoint returns an object with a numbers
field which is an array containing all contact numbers of a Professional Social Network profile.
Example code:
{...}
const PERSONAL_CONTACT_NUMBER_ENDPOINT = 'https://nubela.co/proxycurl/api/contact-api/personal-contact';
const Professional Social Network_PROFILE_URL = 'https://professionalsocialnetwork.com/in/test-phone-number';
const personalContactNumberConfig = {
url: PERSONAL_CONTACT_NUMBER_ENDPOINT,
method: "get",
headers: { Authorization: "Bearer " + process.env.API_KEY },
params: {
Professional Social Network_profile_url: Professional Social Network_PROFILE_URL,
},
};
const getPersonalContactNumber = async () => {
try {
return await axios(personalContactNumberConfig);
} catch (err) {
throw new Error(err);
}
};
const number = await getPersonalContactNumber();
console.log("Personal number:", number.data);
{...}
The above example logs the following response to the console:
Personal number: { numbers: [ '+1123123123' ] }
Summary
B2B work emails are not easily available on Professional Social Network profiles. You need external tools, and most external tools do not work with Professional Social Network profiles programmatically, at scale.
With Proxycurl's Contact API for Professional Social Network profiles, you get to enjoy a fast and reliable experience scaling your applications with fresh and rich data without worrying about compliance or legal issues. Get started now at https://nubela.co/proxycurl/pricing to get a plan that suits your development need.