Unfortunately LinkedIn no longer offers any kind of employee data through their official API. But you can use third-party LinkedIn APIs like Proxycurl (hello! that's us) instead.
It can be as simple as a query to our Employee Listing Endpoint with your specified company, here's a Python example for pulling all Apple employees:
import requests
api_key = 'Your_API_Key_Here'
headers = {'Authorization': 'Bearer ' + api_key}
api_endpoint = 'https://nubela.co/proxycurl/api/linkedin/company/employees/'
params = {
'url': 'https://www.linkedin.com/company/apple/',
}
try:
response = requests.get(api_endpoint, params=params, headers=headers)
print("Status Code:", response.status_code)
print(response.json())
except requests.exceptions.RequestException as e:
print("Error:", e)
That said, want some proof it actually works?
We've prepared a JSON export of:
- A list of 1,000 current Apple employees
- A list of 10 current enriched Apple employees
You can download the two demo lists of current Apple employees here:
(Check your email after submission.)
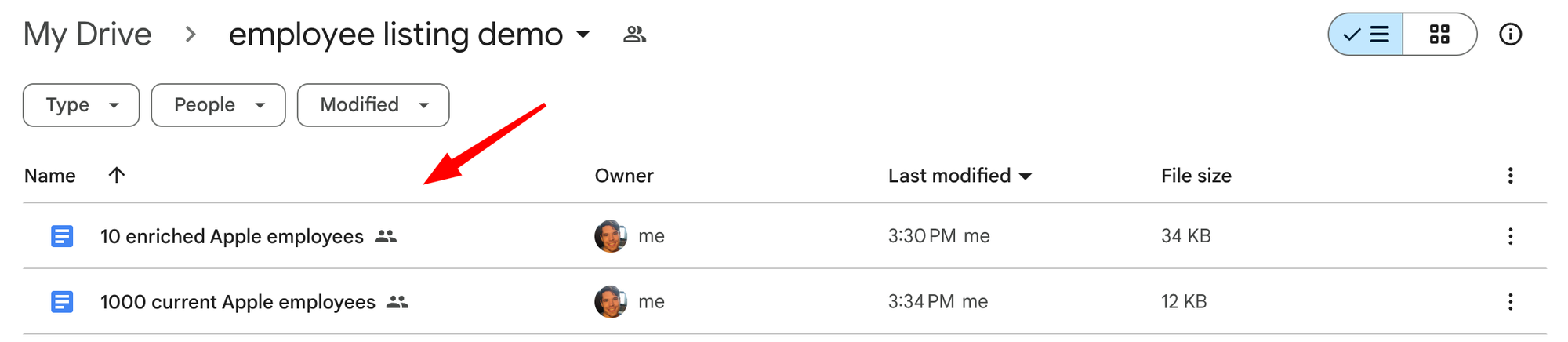
Of course, you could export even more yourself.
Continuing on:
How Proxycurl's API works
Step 1) We scrape data from various sources
The first step to our API is scraping data from various sources. Our primary data source is LinkedIn (we actually sell our public LinkedIn database dump, it's called LinkDB), but we also use additional data sources for enrichment.
We take care of all the headaches involved like dealing with cookies, proxies, CAPTCHAs, LinkedIn accounts, and beyond. There is zero risk of losing your LinkedIn account with a third-party LinkedIn API in comparison to desktop or browser-based LinkedIn data extraction solutions.
Step 2) Data parsing, filtering, and organizing
Profiles are parsed into JSON format, making it easier to organize and filter.
This makes the next step easier (and makes it easier for you to receive and manipulate this B2B data however you like).
Step 3) We offer that data through our API endpoints
Using our exhaustive B2B database - LinkDB, we're able to offer API endpoints like the Employee Listing Endpoint, which allows you to pull a list of employees for any given LinkedIn company URL just as well as the official API would work--if they had it.
How to use Proxycurl's third-party LinkedIn API to pull a list of employees
Step 1) Create a Proxycurl account
The first thing you'll need to do is create your Proxycurl account for free here.
Now, in terms of pricing, we use a credit usage system for billing. For example, it costs 3 credits per every employee returned on our Employee Listing Endpoint, without any additional enrichment parameters enabled (full pricing breakdown is available here).
When you create your account, you'll receive 15 free trial credits with the ability to complete various onboarding tasks for more, and then the ability to further test out our API by topping up as low as $10. It's very cost-effective, even for tight budgets.
Step 2) Set up your environment
There are plenty of different ways to pull data from an API. Pick your language of choice, and run with it.
In my case, I'm familiar with Python, so I use Python. If you need a Python IDE, PyCharm is a good free one.
In our documentation, we even provide a Python example. Using that same example we can pull a list of employees incredibly easily.
Searching for software developers at Apple
Let's say we want to look up "software developer" employees actively working for Apple in the United States.
Here's how we could do that using a bit Python and the Employee Listing Endpoint:
import requests
api_key = 'Your_API_Key_Here'
headers = {'Authorization': 'Bearer ' + api_key}
api_endpoint = 'https://nubela.co/proxycurl/api/linkedin/company/employees/'
params = {
'url': 'https://www.linkedin.com/company/apple/',
'country': 'us',
'enrich_profiles': 'enrich',
'role_search': '(?i)Software Developer',
'page_size': '10',
'employment_status': 'current',
}
try:
response = requests.get(api_endpoint, params=params, headers=headers)
print("Status Code:", response.status_code)
print(response.json())
except requests.exceptions.RequestException as e:
print("Error:", e)
Step 3) Retrieve data from our API
Click run on the Python script above to query our API and...
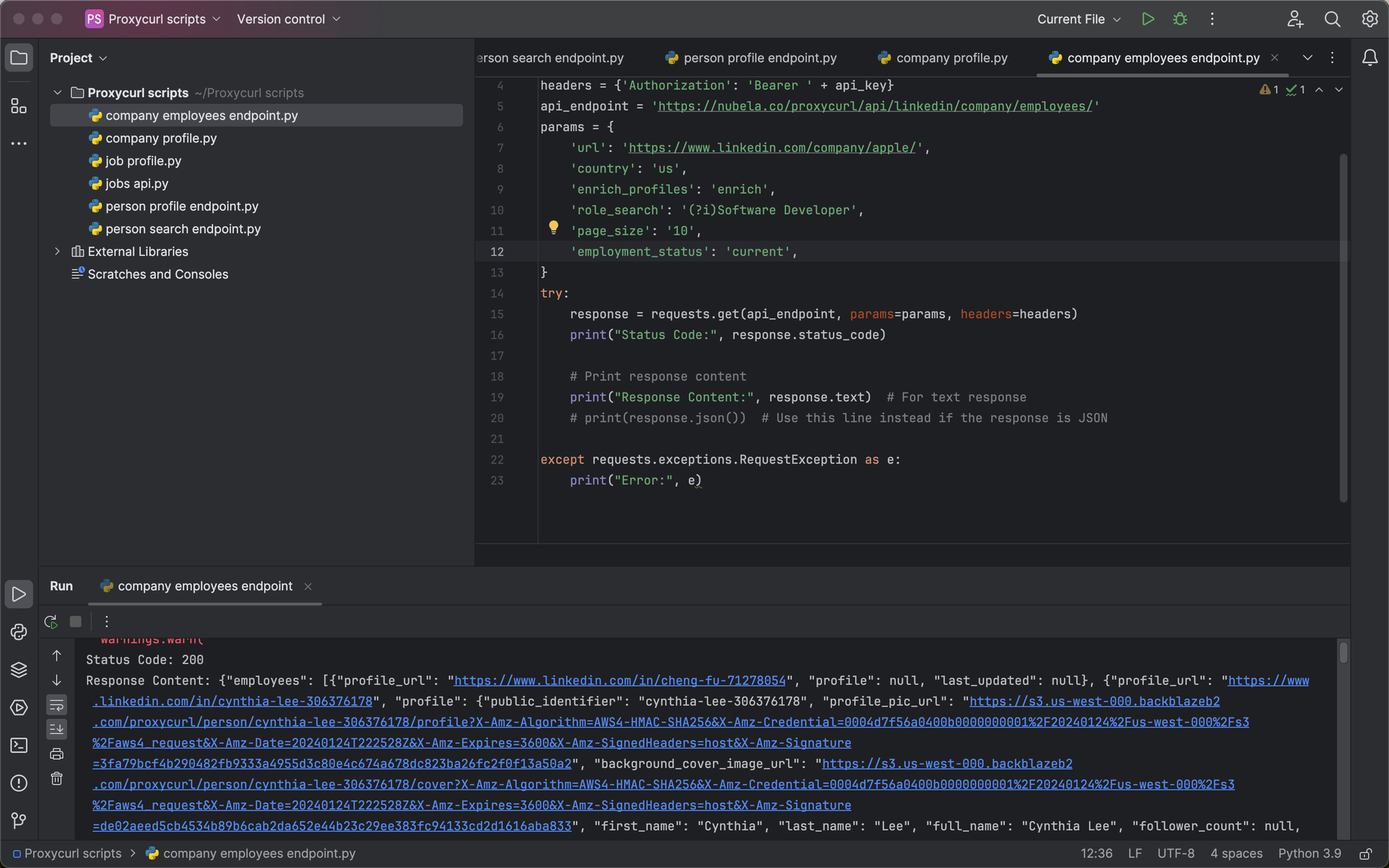
Success! You'll see a list of 10 enriched United States Apple employees currently in the "software developer" role returned.
The immediate page will be 10 contacts due to the enrichment (which is our maximum with the enrichment feature enabled), but with pagination you can navigate through all of the available employees that fit that role.
Can I pull all employees at a given company with the Employee Listing Endpoint?
Yes, the only parameter that's required with this API endpoint is 'url': 'https://www.linkedin.com/company/apple/'
(specifying the company), so if you remove the rest, as shown at the very beginning of this article, you'll get the full list of employees in entirety.
Other possibilities with Proxycurl
Pull all jobs posted by a given company
Being able to search all employees at a given company is nice, but what about being able to pull current job listings for a given company?
We can help out with that too, with our Job Search Endpoint.
Using some simple Python, we could pull all LinkedIn jobs currently posted by Microsoft with the following code:
import requests
api_key = 'Your_API_Key_Here'
headers = {'Authorization': 'Bearer ' + api_key}
api_endpoint = 'https://nubela.co/proxycurl/api/v2/linkedin/company/job'
params = {
'search_id': '1035',
}
try:
response = requests.get(api_endpoint, params=params, headers=headers)
print("Status Code:", response.status_code)
print(response.json())
except requests.exceptions.RequestException as e:
print("Error:", e)
Which would then return a result similar to this, listing all jobs:
Response Content: {"job": [{"company": "Microsoft", "company_url": "https://www.linkedin.com/company/microsoft", "job_title": "Software Engineer I", "job_url": "https://www.linkedin.com/jobs/view/software-engineer-i-at-microsoft-3765124541", "list_date": "2024-01-10", "location": "Bengaluru, Karnataka, India"}, {"company": "Microsoft", "company_url": "https://www.linkedin.com/company/microsoft", "job_title": "Data Analyst", "job_url": "https://www.linkedin.com/jobs/view/data-analyst-at-microsoft-3751276114", "list_date": "2024-01-10", "location": "Hyderabad, Telangana, India"}, {"company": "Microsoft", "company_url": "https://www.linkedin.com/company/microsoft", "job_title": "Software Engineer \u2013 Web", "job_url": "https://www.linkedin.com/jobs/view/software-engineer-%E2%80%93-web-at-microsoft-3770150941", "list_date": "2024-01-14", "location": "Hyderabad, Telangana, India"}, {"company": "Microsoft", "company_url": "https://www.linkedin.com/company/microsoft", "job_title": "Applied Sciences IC2", "job_url": "https://www.linkedin.com/jobs/view/applied-sciences-ic2-at-microsoft-3794668824", "list_date": "2024-01-05", "location": "Bengaluru, Karnataka, India"}, {"company": "Microsoft", "company_url": "https://www.linkedin.com/company/microsoft", "job_title": "Administrative Assistant (contract)", "job_url": "https://www.linkedin.com/jobs/view/administrative-assistant-contract-at-microsoft-3805494445", "list_date": "2024-01-15", "location": "Redmond, WA"}, {"company": "Microsoft", "company_url": "https://www.linkedin.com/company/microsoft", "job_title": "Communications Manager", "job_url": "https://www.linkedin.com/jobs/view/communications-manager-at-microsoft-3782203074", "list_date": "2024-01-05", "location": "Gurgaon, Haryana, India"}, {"company": "Microsoft", "company_url": "https://www.linkedin.com/company/microsoft", "job_title": "Administrative Assistant (contract)", "job_url": "https://www.linkedin.com/jobs/view/administrative-assistant-contract-at-microsoft-3802685788", "list_date": "2024-01-10", "location": "Redmond, WA"}, {"company": "Microsoft", "company_url": "https://www.linkedin.com/company/microsoft", "job_title": "Researcher", "job_url": "https://www.linkedin.com/jobs/view/researcher-at-microsoft-3799970948", "list_date": "2024-01-11", "location": "Bengaluru, Karnataka, India"}, {"company": "Microsoft", "company_url": "https://www.linkedin.com/company/microsoft", "job_title": "Researcher", "job_url": "https://www.linkedin.com/jobs/view/researcher-at-microsoft-3799973649", "list_date": "2024-01-11", "location": "Bengaluru, Karnataka, India"}
Going on, and on, listing all jobs posted unless otherwise filtered with parameters.
We also have a few additional relevant endpoints that could help you out here, such as:
- Job Listing Count Endpoint, which counts the number of jobs posted by a company on LinkedIn
- Job Profile Endpoint, which returns a structured result of a job listing
- Employee Count Endpoint, which counts the number of employees at a given company
Cons of third-party LinkedIn APIs
You'll only get public data
Many people wonder is "scraping LinkedIn legal?" and the short answer is yes, but we have to respect individuals' privacy settings.
This means we won't have quite as much data available as LinkedIn itself, as we only work with publicly available data, but we come close (hundreds of millions of profiles).
Concerns about reliability
You might be concerned about relying on a business or product that relies on another business or product. The main concept after all of a third-party API is scraping data from another source.
What if a new update on said data source wipes out the ability to supply new data overnight?
While I can't speak on every API out there that relies on scraping their data from another source, I can speak on our API-- we've invested significantly in our infrastructure to make sure we're set for years to come. There are zero concerns about reliability when it comes to pulling LinkedIn data from our API for years to come.
Pros of third-party LinkedIn APIs
Way more possibilities
LinkedIn limits you a lot more than a third-party LinkedIn API would. Our goal is to create new solutions for you that should be possible, but aren't.
We offer unique endpoints that allow you to pull all kinds of data that otherwise wouldn't be available officially.
Flawless integration into your systems
Between all of our different API endpoints, we definitely have what you need in terms of LinkedIn data, and we've given you the perfect way to build that data into your systems. We love software engineers.
In comparison to a platform that intentionally makes it difficult to extract data from it; we welcome it, and we love to see all of the interesting projects that are built using the B2B data we provide as a foundation.
Not bad, huh?
You now have practically all of the employee data you could ever possibly need, and you can integrate it according to your needs.
If you haven't already, you can click here to create your account today and receive 15 free credits to try out a few basic actions and get a feel for our API.
Thanks for reading! Here's to fewer limitations and more data!
P.S. Have any questions? Don't hesitate to reach out to us at "[email protected]" for help.
P.P.S. I'm figuring you're probably pretty technically inclined if you're searching for anything related to an API, but we also have an alternative convenient Google Sheets extension named Sapiengraph that'll allow you to conveniently pull the exact same employee quality data into a Google Sheet. You might give it a try.